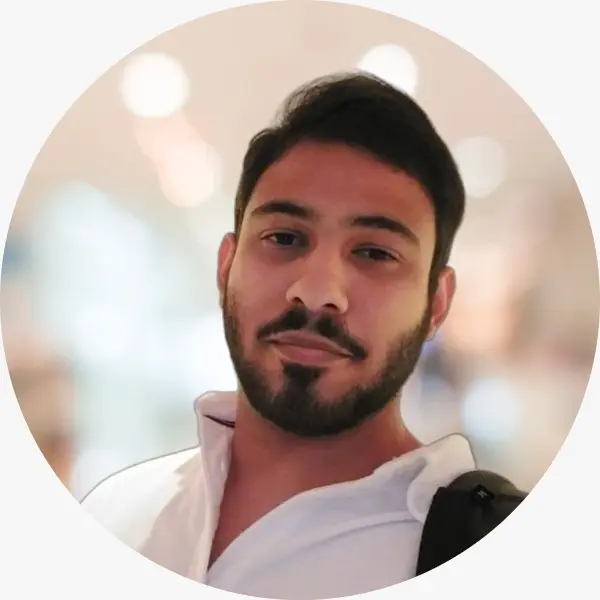
buarki
FollowSite Reliability Engineer, Software Engineer, coffee addicted, traveler
Optimizing Struct Layout and Padding in Practice
April 27, 2025
6 views
Share it
What is Struct Layout?
When working with Go, understanding how structs are laid out in memory is crucial for writing efficient code. Struct layout refers to how fields within a struct are arranged in memory, including any padding that the compiler adds to ensure proper alignment.
In Go, the compiler follows specific rules for struct layout to ensure efficient memory access and proper alignment with the underlying hardware architecture. This process is automatic, but understanding it can help you write more memory-efficient code.
Why is Struct Layout Important?
The way structs are laid out in memory can have significant implications for your application's performance and memory usage. Here's why it matters:
1. Memory Efficiency: Poor struct layout can lead to wasted memory due to padding. The compiler adds padding bytes to ensure that fields are properly aligned, which can sometimes result in significant memory overhead.
2. Cache Utilization: Modern CPUs use cache lines (typically 64 bytes) to fetch data from memory. If your struct fields are scattered due to padding, you might need more cache lines to access the same amount of data.
3. Performance Impact: Accessing memory that isn't properly aligned can lead to performance penalties, as the CPU might need to perform multiple memory accesses to read a single value.
Understanding Padding
Take a look at this one:
type Example struct {
a bool // 1 byte
b int64 // 8 bytes
c bool // 1 byte
}
You might expect this struct to take 10 bytes (1 + 8 + 1), but due to alignment requirements, it actually takes 24 bytes! Here's why:
1. The int64
field needs to be aligned on an 8-byte boundary
2. The compiler adds 7 bytes of padding after the first bool
3. It also adds 7 bytes of padding after the second bool
to maintain alignment for potential subsequent fields
We can optimize this by reordering the fields:
type OptimizedExample struct {
b int64 // 8 bytes
a bool // 1 byte
c bool // 1 byte
}
This optimized version only takes 16 bytes because the bool
fields can share the same padding space. The rule of thumb is: place larger fields first.
Practical Benefits
1. Reduced Memory Usage: In systems where memory is constrained or when dealing with large numbers of structs, proper layout can significantly reduce memory consumption.
2. Better Cache Performance: When structs are properly laid out, more data can fit into a single cache line, reducing cache misses and improving performance.
3. Improved Serialization: When sending structs over the network or storing them on disk, proper layout can reduce the amount of data that needs to be transferred or stored.
Best Practices
Here are some best practices for struct layout in Go:
1. Order fields by size: Place larger fields first, followed by smaller ones.
2. Group related fields: Keep related fields together to improve cache locality.
3. Consider alignment requirements: Be aware of the alignment requirements of different types (e.g., int64 needs 8-byte alignment).
4. Use tools: Leverage tools like viztruct to analyze and optimize your struct layouts.
Practical Benchmark
I've created a simple benchmark to demonstrate the impact of struct layout on performance and memory usage. It compares the two following structs: one with poor layout and one with optimized layout:
// Poor layout - fields ordered by size
type PoorLayout struct {
a bool // 1 byte
b int64 // 8 bytes
c bool // 1 byte
d int32 // 4 bytes
e bool // 1 byte
}
// Optimized layout - larger fields first
type OptimizedLayout struct {
b int64 // 8 bytes
d int32 // 4 bytes
a bool // 1 byte
c bool // 1 byte
e bool // 1 byte
}
The outputs I got from this benchmarks are:
$ go test -bench=. -benchmem
goos: darwin
goarch: amd64
pkg: github.com/buarki/chvv
cpu: VirtualApple @ 2.50GHz
BenchmarkMemoryAllocation/PoorLayout-12 1207 843062 ns/op 32006154 B/op 1 allocs/op
BenchmarkMemoryAllocation/OptimizedLayout-12 2422 447033 ns/op 16007174 B/op 1 allocs/op
BenchmarkFieldAccess/PoorLayout-12 2146 567032 ns/op 0 B/op 0 allocs/op
BenchmarkFieldAccess/OptimizedLayout-12 4645 257307 ns/op 0 B/op 0 allocs/op
PASS
ok github.com/buarki/chvv 5.214s
Note: These benchmarks were run on a VirtualApple CPU (2.50GHz) using Go 1.21 on macOS. The numbers might vary slightly on different architectures, but the relative improvements should be similar.
The results show some interesting insights:
1. Memory Usage: The PoorLayout
struct uses 32 bytes per instance, while the OptimizedLayout
uses only 16 bytes. This means we save 16 bytes per struct instance, which is a 50% reduction in memory usage. For 1 million instances, this translates to 32MB vs 16MB - a massive difference that directly affects your application's memory footprint and garbage collection overhead.
2. Allocation Performance: The optimized version is 47% faster in allocation (447,033 ns/op vs 843,062 ns/op). This improvement comes from reduced memory pressure and better cache utilization during allocation. The CPU can process more optimized structs in the same time frame because they fit better in the cache hierarchy.
3. Field Access Performance: The optimized version shows a 55% improvement in field access speed (257,307 ns/op vs 567,032 ns/op). This significant performance gain comes from better cache locality and reduced cache line misses. Modern CPUs have a hierarchy of caches (L1, L2, L3) with different sizes and speeds:
• L1 Cache: The fastest but smallest (typically 32-64KB per core)
• L2 Cache: Medium speed and size (typically 256KB-1MB per core)
• L3 Cache: The largest but slowest (typically 2-32MB shared)
The optimized layout allows more structs to fit in these caches, reducing the need to fetch data from main memory. When accessing fields in the poor layout, the CPU might need to load multiple cache lines due to padding, while the optimized layout can often fit multiple structs in a single cache line.
The improvements are particularly significant in scenarios where you're dealing with large numbers of structs or performance-critical code paths. The combination of reduced memory usage and improved cache utilization can lead to substantial performance gains in real applications.
Ok, but where does this make any difference? Some practical scenarios where these optimizations matter:
1. Web Servers: A typical web server might handle thousands of concurrent requests, each potentially creating multiple structs for request processing, authentication, and response formatting. For example, if your server processes 10,000 requests per second, each creating 100 structs, that's 1 million structs per second - and the memory savings add up quickly.
2. Data Processing: When processing large datasets, you might load thousands of records into memory. For instance, a CSV file with 100,000 rows, each represented by a struct, would save 1.6MB of memory with optimized layout. This becomes even more significant when dealing with multiple concurrent data processing tasks.
3. Game Development: In game engines, you might have thousands of entities (players, NPCs, items) each represented by structs. The memory savings and performance improvements can be crucial for maintaining smooth gameplay, especially on resource-constrained devices.
4. IoT Devices: On resource-constrained devices, every byte counts. Optimizing struct layouts can help reduce memory usage and improve battery life by reducing the number of memory operations.
In these scenarios, the cumulative effect of struct layout optimization can lead to:
• Reduced memory pressure and fewer garbage collection cycles
• Better cache utilization and faster processing
• Lower resource requirements and better scalability
• Improved battery life on mobile and IoT devices
You can run the benchmark on your machine to see the actual numbers. The results might vary depending on your CPU architecture and Go version, but the relative differences should be similar.
Using Viztruct to Optimize Struct Layout
While you can manually optimize struct layouts, tools like viztruct can help visualize and optimize your struct layouts automatically. These tools can:
1. Show the actual memory layout of your structs
2. Suggest optimal field ordering
3. Calculate padding and alignment
4. Help identify potential memory waste
You can use viztruct through it web app or install it locally:
go install github.com/buarki/viztruct/cmd/viztruct@latest
Conclusion
Understanding and optimizing struct layout is an important aspect of writing efficient Go code. While the compiler handles most alignment automatically, being aware of these concepts can help you write more memory-efficient and performant code, especially in resource-constrained environments or when dealing with large numbers of structs.
Remember that optimization should be guided by profiling and actual performance requirements. Not every struct needs to be perfectly optimized, and there are scenarios where struct padding should be "disabled", like in C networking code where you need precise control over memory layout for protocol headers. However, understanding these concepts will help you make informed decisions when performance matters.